The python tool in Alteryx is a great resource that I often use but it can easily get very cluttered with lots of reusable code that could easily be moved out and imported as a library.
Unfortunately by default you can’t just use the standard import statement since the location of your workflow at runtime is not in pythons path so it doesn’t search for libraries there.
Take this example where I have a python file called carrierpy.py located in the same directory as my Alteryx workflow. The file contains a simple function to return the dates for the start and end of the previous two week period.
import datetime
def last_week():
today = datetime.datetime.today()
weekday = today.weekday()
start_delta = datetime.timedelta(days=weekday, weeks=2)
start_of_week = today - start_delta
end_of_week = start_of_week + datetime.timedelta(days=13)
return (start_of_week, end_of_week)
If you try to load this library from the python tool in Alteryx (import carrierpy as cr) you’ll see this error. The python tool cannot find the library as it’s not searching the current workflow path.
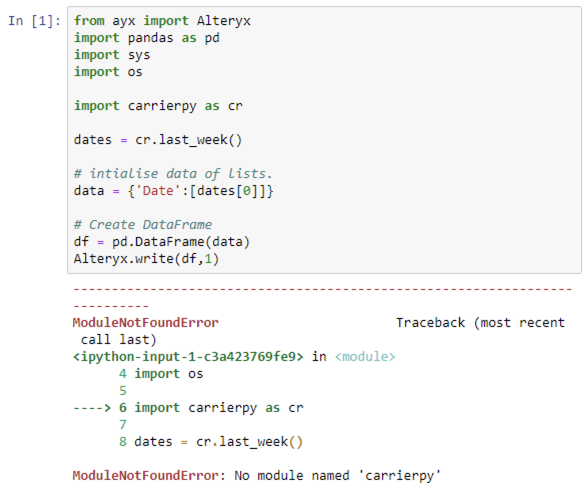
Standard ways to access the current working directory also cannot be used as they return the path to the temp location used by the Jupyter notebook, not the directory the Alteryx workflow is saved in.
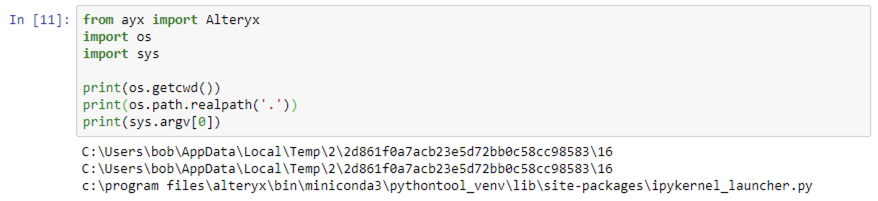
Workflow Constant Solution
To allow this to work we need to use the Alteryx module that we import at the start of every python tool.
In the python tool add the following code at top of the notebook to read the current path from the Alteryx library and add this to to sys.path. Then we can load modules from the local directory without issues.
from ayx import Alteryx
import pandas as pd
import sys
# get the path of the Workflow Directory
path = Alteryx.getWorkflowConstant('Engine.WorkflowDirectory')
# append this path to the python sys.path
sys.path.append(path)
# import from current directory
import carrierpy as cr
dates = cr.last_week()
# initialise data of lists.
data = {'Date':[dates[0]]}
# Create DataFrame
df = pd.DataFrame(data)
Alteryx.write(df,1)
Sure enough this works and if we run the Jupyter notebook we see the correct data printed (make sure you run the entire workflow first otherwise the input path will not be populated and cached).
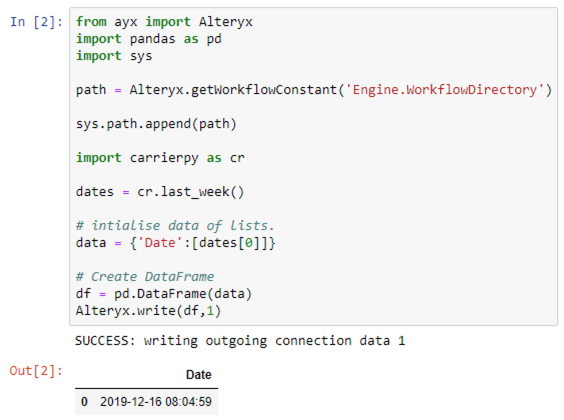
In the Alteryx workflow messages we now see the data is correctly passed out of the python tool.
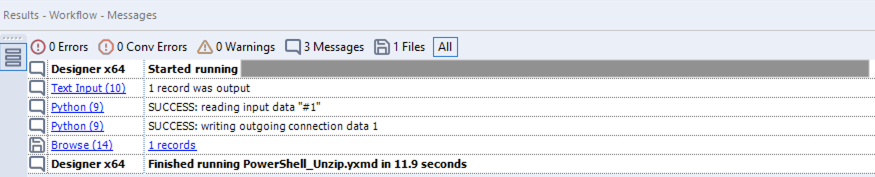
The browse tool also shows the date being output from the python tool 🐍
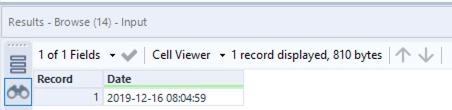
Now you can create your own libraries of often used python functions and import them into your workflows as required ♻️