Ever wanted to automate a process involving a vendor website and come across this. Me too π
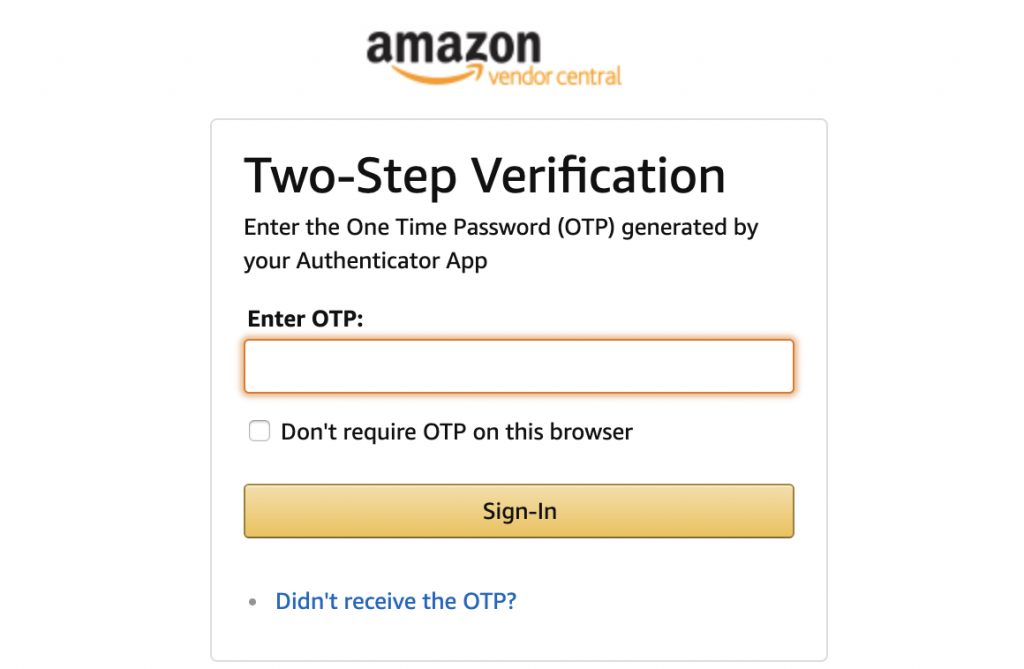
One time passwords (aka MFA, 2FA, or two factor authentication as I’ll say from here on) are something everyone should use and are great for security, not so great for automation.
Until recently I thought this was hard to overcome without some super hacky solution but if you’re using selenium with python the solution is at hand and really easy to implement.
This solution works if you’re using an authenticator app like Google Authenticator that generates time based tokens that change every 60 seconds (which I recommend over SMS due to the sim swap security issue).
Using pyotp
The pyotp library handles creating new one time tokens. To enable the creating of new tokens we must know the secret key that we use when initially setting up the OTP.
When enabling 2FA you are usually prompted to scan a QR code. In reality this is simply a convenient way to enter the secret π, since the code is just the secret key encoded as a QR code.
As well as the QR code thereβs always an option to enter the key manually, use this option and copy the key to a secure location. The key will usually be a long string of letters and numbers like E7XCRPCJABXKM575P3EIVNKYVG3DBRZD.
Note that if someone else gets your secret π they will also be able to generate your tokens so please look after them!
Authenticator apps like Google Authenticator work offline by generating tokens based on the secret key and a time stamp. Using the same π we can generate the same tokens in python.
The Code
First import the library and initialise with your secret π
from pyotp import *
# initialise with your secret key
totp = TOTP("E7XCRPCJABXKM575P3EIVNKYVG3DBRZD")
When you want to create a token simply call this function.
token = totp.now()
Now you can use the send_keys function in selenium to populate the 2FA field in the web application.
# find OTP element on page and send token
e = driver.find_elements(By.ID, "auth-mfa-otpcode"))
e.send_keys(token)
Testing
To test the tokens are working you can use the site below. Type anything as the username and it will give you the secret π to use in your script.
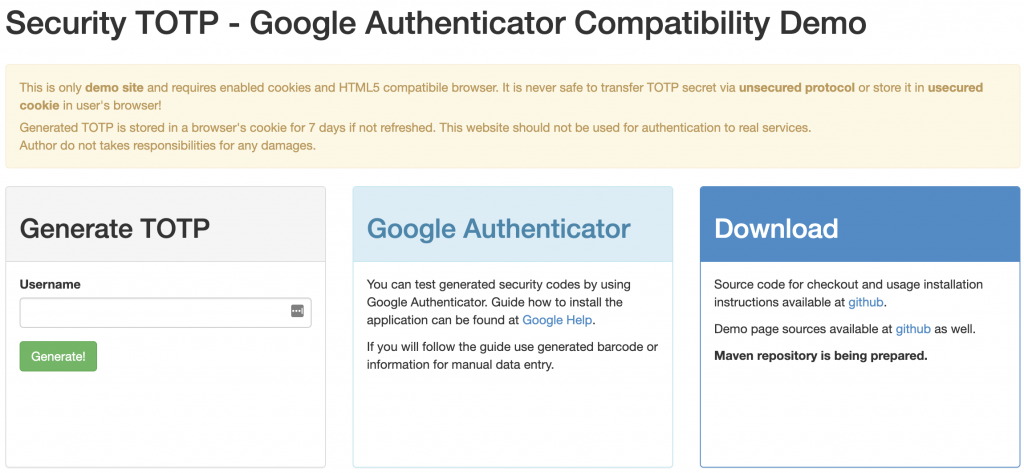
Integrations
This solution could also be integrated into other applications by either embedded a small python script into the flow (using Alteryx or KNIME for example) or alternatively by creating a private API using Flask so any application supporting APIs can simply get the token via a simple API call.
You can now access any site protected by 2FA π