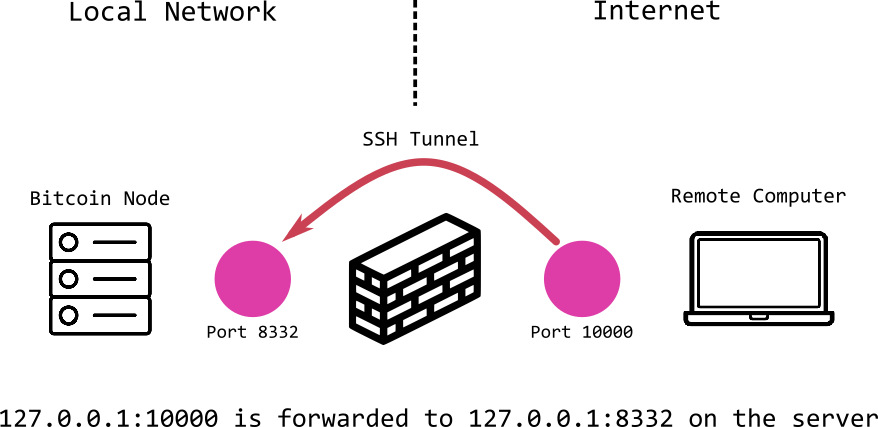
If you’re running a Bitcoin full node and want to run RPC commands against the Bitcoin client from a remote machine the easiest and safest way to do this is using Port Forwarding over an SSH connection.
What is Port Forwarding used for?
Secure access to a port that is otherwise not listening on a public network interface. This is common with database servers like MySQL.
Port Forwarding – https://docs.termius.com/termius-handbook/port-forwarding
Encryption for for services that may not natively use encrypted connections.
This also gives you the flexibility of using Python (or another language) from the remote machine without having to install it on the Bitcoin node.
In my case I’m going to use Python in a Juptyer Notebook to query the node using Termius as the SSH client.
The Bitcoin node is running on my local network and does not accept RPC commands from the internet, but using port forwarding I’ll be able to query it from my laptop from any location.
Install an SSH Client
On Windows I recommend Termius as it’s very easy to use and has a nice graphical interface (it’s also available for Mac, Linux, Android and iOS) but you could use any SSH client (PUTTY for example).
First create an SSH host to the Bitcoin full node.
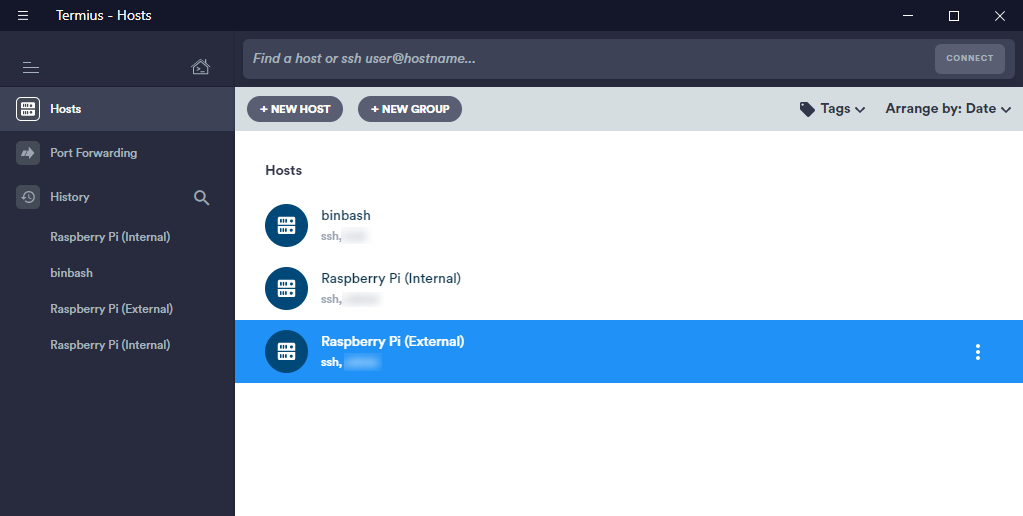
Then create the forwarded port. On your local machine you can select any port that’s not in use, in my case I use port 10000.
When I connect to my local machine on port 10000 the port is then securely forwarded to the remote machine on port 8332, which is the port the Bitcoin RPC server listens on by default.
So 127.0.0.1:10000 becomes BITCOIN_NODE:8332
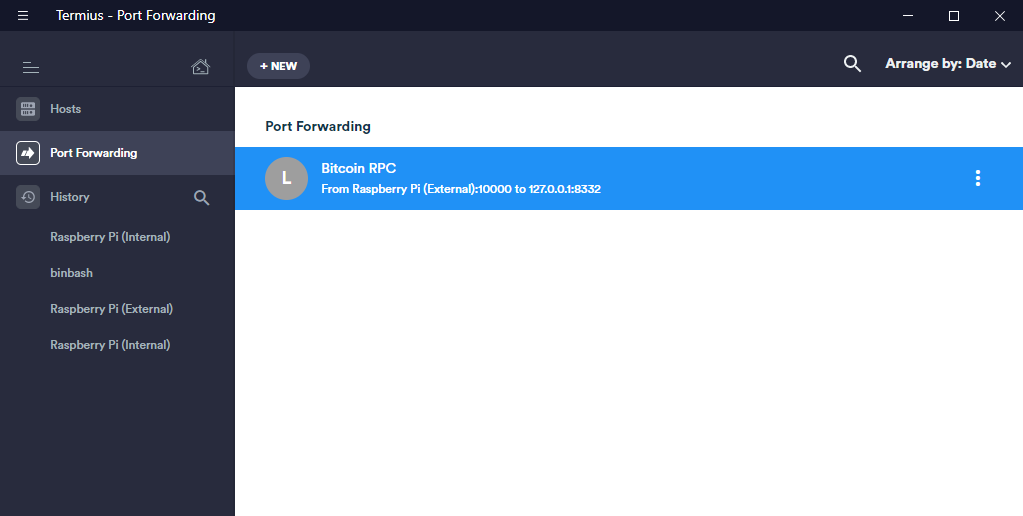
The configuration page should look something like this.
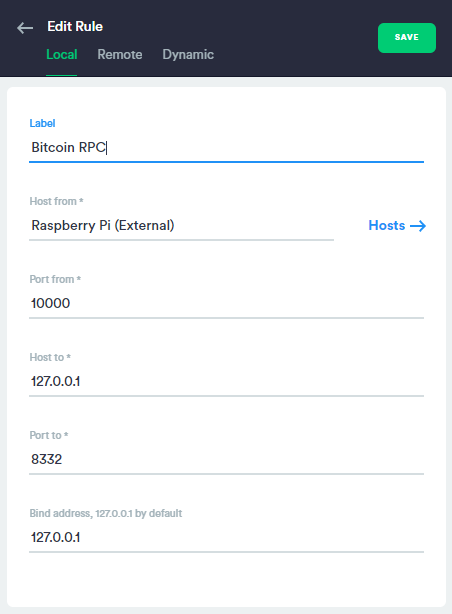
Open the port by clicking connect.
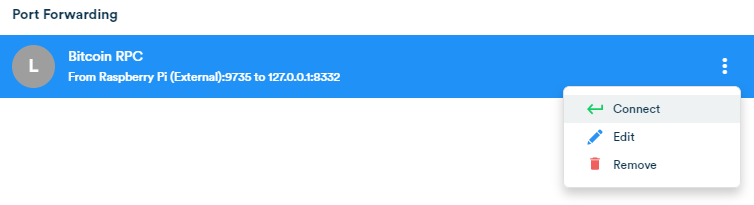
Python Bitcoin Library
To use python with your Bitcoin node use the python-bitcoinrpc library. To install simply use:
pip install python-bitcoinrpc
Next get the rpcuser and password you added to your bitcoin.conf file
rpcuser=thisismyuser rpcpassword=DONT_USE_THIS_YOU_WILL_GET_ROBBED_ijfr84ur84uof94ur9r4
Once installed create a connection to the node using these credentials. The IP will always be locahost (127.0.0.1) and the port is the same port you used for the forwarding, 10000 in my case.
from bitcoinrpc.authproxy import AuthServiceProxy, JSONRPCException
USERNAME = ******
PASSWORD = ******
IP = "127.0.0.1:10000"
rpc_connection = AuthServiceProxy("http://{}:{}@{}".format(USERNAME, PASSWORD, IP), timeout = 500)
One connected we can query the node using regular RPC commands. Here I get the last 10 blocks and return the block height, timestamp, number of transactions in the block, difficulty and nonce.
bci = rpc_connection.getblockchaininfo()
maxBlock = bci["blocks"]
for i in range(maxBlock,maxBlock-10,-1):
bbh = rpc_connection.getblockhash(i)
bh = rpc_connection.getblockheader(bbh)
print(bh["height"],bh["time"],bh["nTx"],bh["difficulty"],bh["nonce"])
Command output:
597577 1570042322 2866 12759819404408.79 872368408 597576 1570041887 2921 12759819404408.79 2413129693 597575 1570041233 3406 12759819404408.79 2989319068 597574 1570039252 2884 12759819404408.79 3248003543 597573 1570038909 3061 12759819404408.79 259424928 ...
This command returns the network statistics of the node.
net = rpc_connection.getnettotals()
print(net)
{'totalbytesrecv': 9043069394, 'totalbytessent': 83507300429, 'timemillis': 1570047435410, 'uploadtarget': {'timeframe': 86400, 'target': 5242880000, 'target_reached': False, 'serve_historical_blocks': True, 'bytes_left_in_cycle': 3230191636, 'time_left_in_cycle': 55100}}
For a full list of the currently available API calls see the Bitcoin Developer Reference.